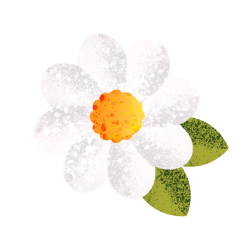
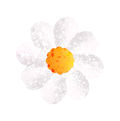
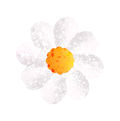
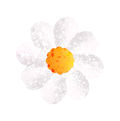
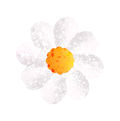
How to Handle API Responses
Steps to Understand API Responses and How to Handle Them
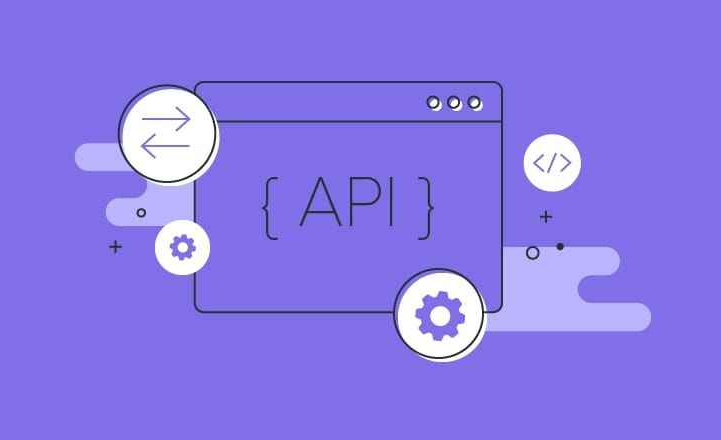
So, since we talked in previous article about making requests to the API from the front end, let’s now focus on how to handle the responses we get back.
When we send a request, we definitely receive a response, and the question is: how do we identify and work with it? That’s the point.
First : Status
Every response comes with a status, just like when you go shopping and they tell you if the item is available or not. In this case, the response is indicated by a specific code known as the Status. So, what are these response statuses?
There are many, but the ones we frequently use are few, usually 200, 400, 404, and 500. You will definitely come across them!
Quick Overview of Important Status Codes:
- 200: Everything is fine; the request was successful.
- 400: There’s a problem; your request might be incorrect.
- 404: The page is not found.
- 500: There’s a server error.
How to Handle the Response
After we receive the response, the first step is to check the status. Then, based on the status, we take specific actions.
- If the status is 200: This means the data was retrieved successfully. Here, we should take the data from the response and display it or save it.
- If the status is 404: We show the user a "Page Not Found" message.
- If the status is 400: We check the error message and inform the user that there is an issue based on what the backend tells us.
Using Axios Interceptors
Now, let’s talk about something cool called Axios Interceptors. This feature is very useful because it allows you to manipulate requests or responses before they are processed.
How to Use Them?
- Request Interceptors: You can use these to add a token or modify headers before sending the request. For example:
axios.interceptors.request.use(config => {
config.headers['Authorization'] = `Bearer ${token}`;
return config;
});
- Response Interceptors: You can use these to handle responses. For example, if you want to log errors:
axios.interceptors.response.use( response => { return response; // If everything is okay }, error => { console.error('Error:', error.response.status); return Promise.reject(error); // If there’s an error } );
Summary
Handling API responses is really important, and we need to know how to deal with status codes. With Axios Interceptors, we can simplify things and add some flexibility to our operations.