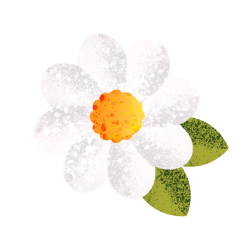
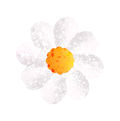
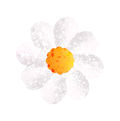
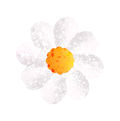
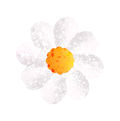
API Integration Using Axios
Some Basics to Consider for Effective API Integration
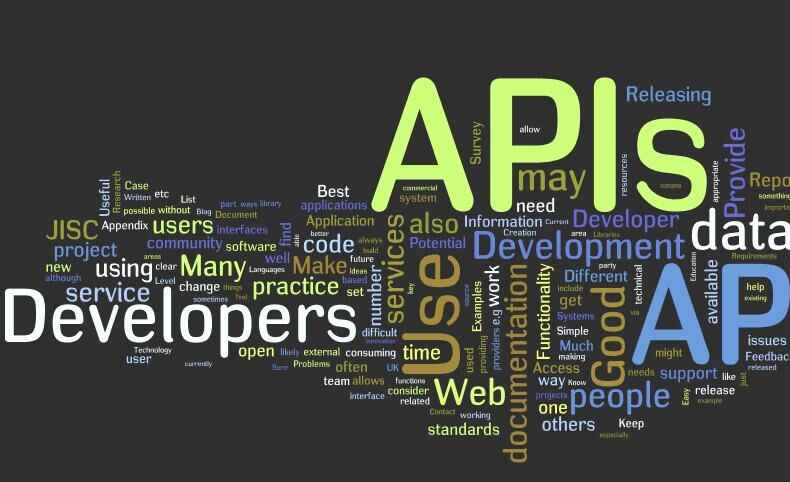
When you're dealing with data from the back-end and using a library like Axios, there are a few things you need to keep in mind to work with the API correctly. In this article, I'll break down the flow simply so you can understand how to use Axios.
First Thing: Think About the API
When we think about any API, there are a few things we need to know:
- URL: The source from which we're fetching data.
- Method: The type of operation we're performing (GET, POST, PUT, DELETE).
- Parameters: The values we're sending or need to use.
The URL
The URL usually splits into two parts:
- Base URL: This is the main link from the back-end. For example:
www.example.com/
- Endpoint: The continuation of the URL that specifies the operation you need. For example:
/user
The Method
The method is the type of operation you're going to do:
- GET: To fetch data.
- POST: To save new data.
- PUT: To modify existing data.
- DELETE: To remove data.
The Parameters
The parameters we send can be divided into four types:
- Query Params: These are the variables that come after the question mark
?
in the URL, separated by&
. Example:
https://www.example.com/products?page=2&search=shirt
- Path Params: These are part of the URL itself. Example:
https://www.example.com/product/123
Here, 123
is the product_id
.
- Body Params: These are the values sent within the body, usually formatted as JSON with methods like POST or PUT.
- Header Params: These contain data like tokens or language. Example:
headers: {'local':'ar', 'authorization':'Bearer token'}
Applying the Examples Using Axios
Example 1: Fetching Product Information with Search and Pagination
var searchVal = ""
var pageVal = ""
var token = ""
axios.get('https://www.example.com/products', {
headers: {'local':'ar', 'authorization': token},
params: { search: searchVal, page: pageVal }
})
Example 2: Updating Product Data with Path, Body, and Header
var body = { name: 'New Product Name', category: 'new-category-id' } axios.put('https://www.example.com/product/1', body, { headers: {'local':'ar', 'authorization': token} })
Why Are These Examples Important?
I wrote this article for anyone wanting to start working with APIs using Axios from a front-end perspective. Understanding these basics will help you interact with the API smoothly without facing issues.